编辑推荐: |
本文来自于cnblogs,介绍了如何创建springBoot项目,数据库,实体类和创建Dao层代码,
创建XML文件代码, 创建Dao层测试类等。 |
|
1 版本说明
springboot:2.0
jdk:1.8

2 创建springBoot项目
创建项目时勾选必要web,MySQL,mybatis相关依赖
创建完成后再pom文件中添加自动部署、lombok相关依赖
2.1 编写测试类
技巧01:项目创建成功后先将mybatis和mysql相关的jar注释掉,在到控制层中创建一个简单的测试类来确认项目是否创建成功
package cn.test.demo.mybatis
_ demo02.controller;
import org.springframework.web.bind.annotation.
GetMapping;
import org.springframework. web.bind.annotation.
RequestMapping;
import org.springframework. web.bind.annotation.
RestController;
/**
* @author 王杨帅
* @create 2018-04-08 7:53
* @desc 控制层测试类
**/
@RestController
@RequestMapping (value = "/test")
public class TestController {
@GetMapping (value = "/test01")
public String test01() {
return "HELLO BOY";
}
} |
2.2 配置文件信息
server.port=9999
server.servlet.path=/dev
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql://127.0.0.1:3306 /testdemo
? useUnicode = true & characterEncoding =
UTF-8 && useSSL = false
jdbc.username=root
jdbc.password=root
mabatis_config_ file = mybatis-config.xml
mapper_path =/mapper/**.xml
entity_package = cn.test.demo.mybatis_demo02.entity
|
3 创建数据库
根据springboot的配置信息,创建一个数据库;并在数据库中创建一个表
技巧01:必须在springboot配置文件中配置的那个数据库中进行数据表的创建
/*
Navicat MySQL Data Transfer
Source Server : mysql5.4
Source Server Version : 50540
Source Host : localhost:3306
Source Database : testdemo
Target Server Type : MYSQL
Target Server Version : 50540
File Encoding : 65001
Date: 2018-04-08 13:33:08
*/
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for `tb_area`
-- ----------------------------
DROP TABLE IF EXISTS `tb_area`;
CREATE TABLE `tb_area` (
`area_id` int(2) NOT NULL AUTO_INCREMENT,
`area_name` varchar(200) NOT NULL,
`priority` int(2) NOT NULL DEFAULT '0',
`create_time` datetime NOT NULL,
`last_edit_time` datetime NOT NULL,
PRIMARY KEY (`area_id`)
) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8mb4;
-- ----------------------------
-- Records of tb_area
-- ----------------------------
INSERT INTO `tb_area` VALUES ('1', '东苑', '0',
'2018-03-07 08:50:37', '2018-04-08 13:28:58');
INSERT INTO `tb_area` VALUES ('2', '南苑', '1',
'2018-04-08 09:44:48', '2018-04-08 09:44:48');
|
4 创建实体类
技巧01:可以利用mybatis提供的生成器来自动生成entity、dao、xml、service、controller对应的代码
技巧02:在编写代码时可以开启代码提示
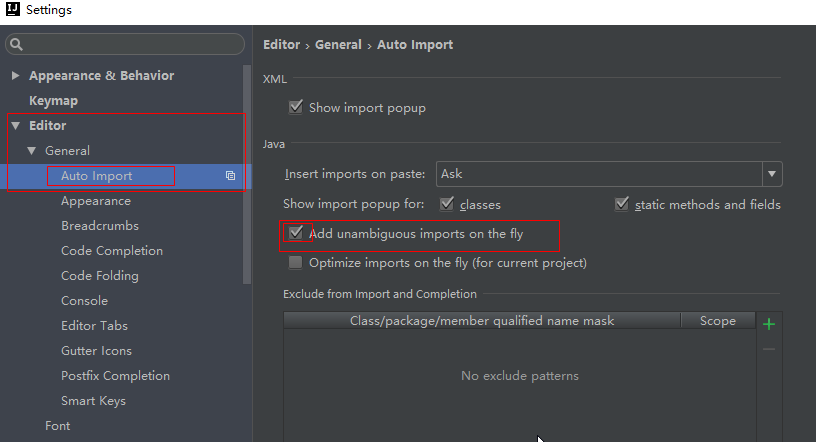
package cn.test.demo
.mybatis_demo02.entity;
import java.util.Date;
/**
* @author 王杨帅
* @create 2018-04-08 8:40
* @desc
**/
public class Area {
// @TableId (value="area_id", type=
IdType.AUTO)
private Integer areaId;
// @TableField ("area_name")
private String areaName;
private Integer priority;
// @TableField ("create_time")
private Date createTime;
// @TableField ("last_edit_time")
private Date lastEditTime;
public Integer getAreaId () {
return areaId;
}
public void setAreaId (Integer areaId) {
this.areaId = areaId;
}
public String getAreaName () {
return areaName;
}
public void setAreaName (String areaName) {
this.areaName = areaName;
}
public Integer getPriority () {
return priority;
}
public void setPriority (Integer priority) {
this.priority = priority;
}
public Date getCreateTime () {
return createTime;
}
public void setCreateTime (Date createTime)
{
this.createTime = createTime;
}
public Date getLastEditTime () {
return lastEditTime;
}
public void setLastEditTime (Date lastEditTime)
{
this.lastEditTime = lastEditTime;
}
@Override
public String toString () {
return "Area {" +
"areaId =" + areaId +
", areaName ='" + areaName + '\''
+
", priority =" + priority +
", createTime
}
} |
5 配置部分
5.1 POM配置
保证pom.xml文件中有mybatis、mysql、mysql驱动这三个对应的依赖
<!--mysql数据库--> <dependency>
<groupId> mysql </groupId>
<artifactId> mysql-connector-java </artifactId>
<scope> runtime </scope> </dependency>
<!--数据库连接池--> <dependency>
<groupId> com.mchange </groupId>
<artifactId> c3p0 </artifactId>
<version> 0.9.5.2 </version> </dependency>
<!--mybatis--> <dependency>
<groupId> org.mybatis.spring.boot </groupId>
<artifactId> mybatis-spring-boot-starter
</artifactId>
<version> 1.3.2 </version> </dependency> |
5.2 mybatis相关配置
在ersources文件夹中创建一个mybatis-config.xml文件用来mybatis相关的配置
技巧01:在项目启动的时候会自动加载resources中的文件进行全局的配置,所有mybatis的配置文件也会被加载
5.2.1 <setting name="useGeneratedKeys"
value="true"/>
开启JDBC的getGeneratedKeys获取数据库自增主键,即:当我们新增一条数据时会自动设置主键值
5.2.2 <setting name="useColumnLabel"
value="ture" />
使用列标签替换列别名
5.2.3 <setting name="mapUnderscoreToCamelCase"
value="true" />
开启驼峰转换,即:数据库中的字段名全部是小写和下划线组成,实体类中的属性全部使用驼峰原则时他们之间就会是一一对应的关系
< ?xml version="1.0"
encoding="UTF-8"? >
<!DOCTYPE configuration
PUBLIC "-// mybatis.org // DTD Config 3.0
// EN"
"http:// mybatis.org/ dtd/ mybatis-3-config.dtd"
>
<configuration> <settings>
<!--使用JDBC的 getGeneratedKeys 获取数据库自增主键-->
<setting name = " useGeneratedKeys "
value ="true"/> <!--使用列标签替换列别名-->
<setting name = "useColumnLabel"
valu e= "ture" /> <!--开启驼峰转换-->
<setting name = "mapUnderscoreToCamelCase"
value = "true" /> </settings>
</configuration> |
5.3 DataSource配置和SqlSessionFactory配置
由于我们使用c3p0作为数据库连接池,所以我们在配置DataSource直接对成c3p0的连接信息进行配置即可
5.3.1 创建一个DataSourceConfiguration类来配置DataSource
注意:类名可以随便取
技巧01:在启动类同一级别或启动类下面级别的地方创建一个config包来存放java配置类
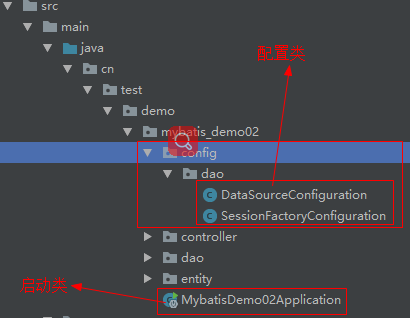
技巧02:java配置类需要在类上添加@Configuration注解来指明该类是一个配置类
技巧03:DataSourceConfiguration配置类说明
生成一个DataSource实例并注入到Bean容器中
技巧01:向数据库连接信息,我们可以把它放到springboot的全局配置文件中去,然后在需要用到的地方通过@Value注解来获取获取,这样就可以做到一改全改的作用
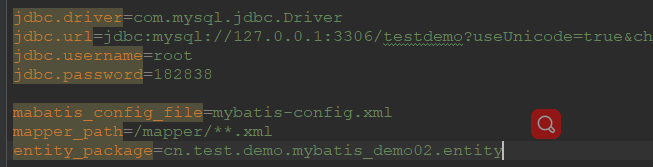
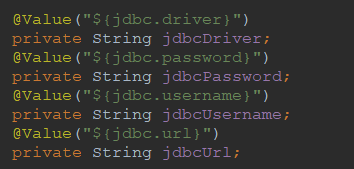
技巧02:DataSource配置类上还需要利用@MapperScan注解来配置mapper映射器的扫描路径,例如:
@MapperScan
("cn.test.demo.mybatis_demo02.dao")
// mapper映射器的扫描路径 |
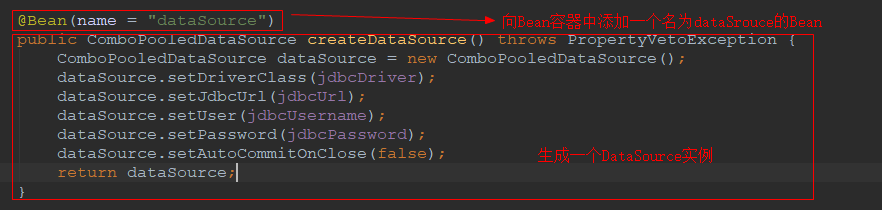
package cn.test.demo.mybatis_demo02.config.dao;
import com.mchange.v2.c3p0.ComboPooledDataSource;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.sql.CommonDataSource;
import java.beans.PropertyVetoException;
/**
* @author 王杨帅
* @create 2018-04-08 8:09
* @desc DataSource配置
**/
@Configuration
@MapperScan("cn.test.demo.mybatis_demo02.dao")
// mapper映射器的扫描路径
public class DataSourceConfiguration {
@Value("${jdbc.driver}")
private String jdbcDriver;
@Value("${jdbc.password}")
private String jdbcPassword;
@Value("${jdbc.username}")
private String jdbcUsername;
@Value("${jdbc.url}")
private String jdbcUrl;
@Bean(name = "dataSource")
public ComboPooledDataSource createDataSource()
throws PropertyVetoException {
ComboPooledDataSource dataSource = new ComboPooledDataSource();
dataSource.setDriverClass(jdbcDriver);
dataSource.setJdbcUrl(jdbcUrl);
dataSource.setUser(jdbcUsername);
dataSource.setPassword(jdbcPassword);
dataSource.setAutoCommitOnClose(false); // 关闭连接后不会自动进行事务提交
return dataSource;
}
} |
5.3.2 创建一个crateSqlSessionFactoryBean类来配置SqlSessionFactory
注意:类名可以随便取
技巧01:java配置类需要在类上添加@Configuration注解来指明该类是一个配置类
技巧02:在配置SqlSessionFactoryBean时需要用到DataSrouce实例,所以需要在配置SqlSessionFactoryBean的配置类依赖注入DataSrouce实例,例如:
@Autowired
@Qualifier("dataSource")
private DataSource dataSource; |
技巧03:配置说明
SqlSessionFactoryBean并注入到Bean容器中
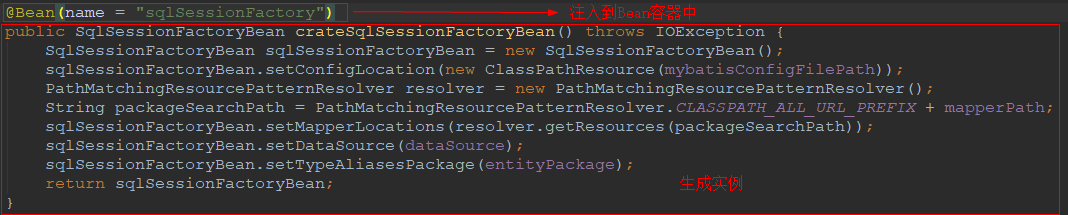
package cn.test.demo.mybatis
_ demo02.config.dao;
import org.apache.ibatis.session. SqlSessionFactory;
import org.mybatis.spring .SqlSessionFactoryBean;
import org.mybatis.spring.annotation .MapperScan;
import org.springframework .beans.factory.annotation.Autowired;
import org.springframework .beans.factory.annotation.Qualifier;
import org.springframework .beans.factory.annotation.Value;
import org.springframework .context.annotation.Bean;
import org.springframework .context.annotation.Configuration;
import org.springframework .core.io.ClassPathResource;
import org.springframework .core.io.support.PathMatchingResourcePatternResolver;
import javax.sql .DataSource;
import java.io .IOException;
/**
* @author 王杨帅
* @create 2018-04-08 8:22
* @desc SessionFactory配置类
**/
@Configuration
//@MapperScan
public class Session FactoryConfiguration {
@Value (" ${mabatis_config_file} ")
private String mybatis ConfigFilePath;
@Value( "${mapper_path}")
private String mapperPath;
@Value( "${entity_package}")
private String entityPackage;
@Autowired
@Qualifier("dataSource")
private DataSource dataSource;
@Bean( name = "sqlSessionFactory")
public SqlSession FactoryBean crateSqlSession
FactoryBean () throws IOException {
SqlSession FactoryBean sqlSession FactoryBean
= new SqlSessionFactoryBean(); // 创建SqlSession
FactoryBean实例
sqlSession FactoryBean.setConfigLocation (new
ClassPathResource ( mybatisConfigFilePath ));
// 扫描mybatis配置文件
// 设置 mappe r映射器对应的XML文件的扫描路径
PathMatching ResourcePatternResolver resolver
= new PathMatchingResource PatternResolver ();
String packageSearch Path = PathMatchingResourcePatternResolver
.CLASSPATH_ALL_URL_PREFIX + mapperPath ;
sqlSessionFactoryBean .setMapperLocations (resolver.getResources
(packageSearchPath));
// 设置数据库连接信息
sqlSessionFactoryBean.setDataSource (dataSource);
// 设置实体类扫描路径
sqlSessionFactoryBean.setTypeAliasesPackage (entityPackage);
return sqlSession FactoryBean;
} |
将扫描路径配置到springboot的全局配置配置文件中,然后利用@Value去获取即可,这样既可以实现一改全改的效果;例如:

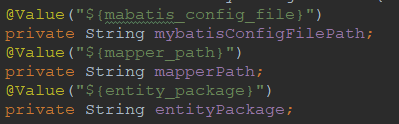
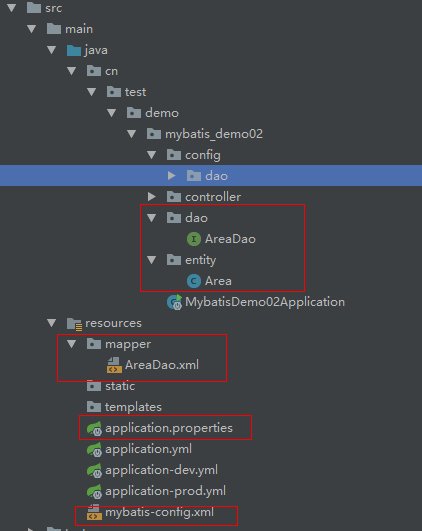
@Autowired进行依赖注入的时候可以利用@Qualifier指定名字,例如:
@Autowired
@Qualifier("dataSource")
private DataSource dataSource; |
6 创建Dao层代码
Dao层在使用mybatis实现时也叫作Mapper映射器,其实Mapper映射器就是一个接口而已
package cn.test.demo.mybatis_demo02.dao;
import cn.test.demo.mybatis_demo02.entity.Area;
import com.sun.applet2.preloader.event.InitEvent;
import java.util.List;
/**
* @author 王杨帅
* @create 2018-04-08 8:42
* @desc
**/
public interface AreaDao {
List<Area> queryArea();
Area queryById(Integer areaId);
Integer insertArea(Area area);
Integer updateArea(Area area);
Integer deleteArea(Integer areaId);
} |
7 创建XML文件代码
一个XML文件和一个Mapper映射器对应,具体这么对应请参见mybatis先关博文
<?xml version="1.0"
encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "- // mybatis.org
// DTD Mapper 3.0 // EN" "http:// mybatis.org
/dtd /mybatis-3 - mapper.dtd">
<mapper namespace = "cn.test.demo.mybatis
_ demo02.dao.AreaDao">
<!-- 通用查询映射结果 -->
<!--<resultMap id = "BaseResultMap"
type = "org.ibase4j.entity.TbArea">-->
<!--<id column = "area_id" property
="areaId" />-->
<!--<result column = "area_name"
property="areaName" />-->
<!--<result column = "priority"
property="priority" />-->
<!--<result column = "create_time"
property="createTime" />-->
<!--<result column = "last_edit_time"
property="lastEditTime" />-->
<!--</resultMap >-->
<select id = "queryArea" resultType
= "cn.test.demo.mybatis _ demo02.entity.Area">
SELECT
*
FROM
tb_area
ORDER BY
priority
DESC
</select>
<select id ="queryById" resultType
="cn.test.demo.mybatis _demo02 .entity .Area">
SELECT
*
FROM
tb_area
WHERE
area_id = #{areaId}
</select>
<insert id ="insertArea"
useGeneratedKeys ="true" keyProperty
="areaId" keyColumn ="area_id"
parameterType ="cn.test.demo.mybatis _demo02.entity.Area">
INSERT INTO
tb_area (area_name, priority, create_time, last_edit_time)
VALUES
( #{areaName}, #{priority}, #{createTime}, #{lastEditTime})
</insert>
<update id ="updateArea" parameterType
="cn.test. demo.mybatis _demo02. entity.Area">
UPDATE tb_area
<set>
<if test ="areaName!=null" >area_name
=#{areaName},</if>
<if test ="priority!=null" >priority
=#{priority},</if>
<if test ="lastEditTime! = null">last_edit_time
=#{lastEditTime}</if>
</set>
WHERE
area_id = #{areaId}
</update>
<delete id ="deleteArea">
DELETE FROM
tb_area
WHERE
area_id = #{areaId}
</delete>
</mapper> |
8 创建Dao层测试类
坑01:在测试类中进行依赖注入时可能会报错,报错信息为:找不到相关的Bean
原因:IDEA是一个比较智能的编辑器,所以在进行依赖注入的时候就会检查需要注入的Bean在容器中是否存在,如果不存在就会报出这样的错误;但是由于IDEA的该功能还不够完善所以即使Bean容器有对应的Bean也会报出找不到相关Bean的错误
解决办法:将IDEA这个功能的报错级别调低,调成warning即可,例如:
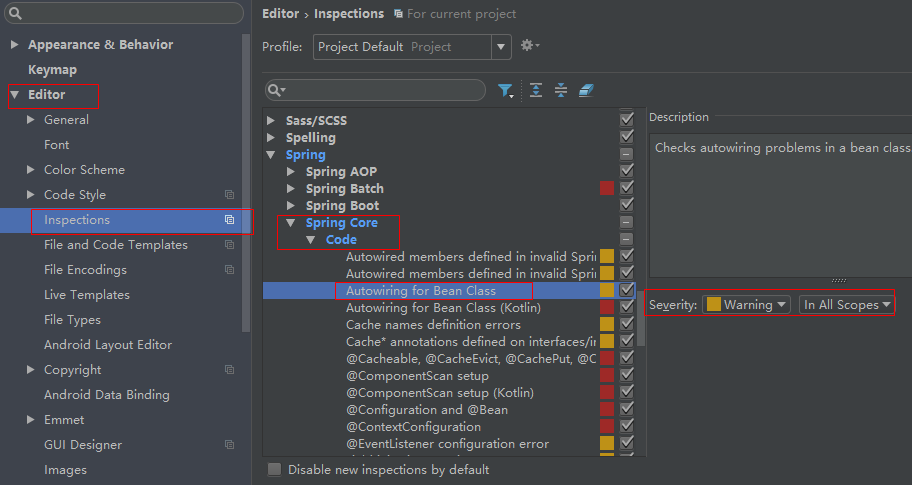
package cn.test.demo.mybatis_
demo02.dao;
import cn.test.demo.mybatis_ demo02.entity.Area;
import lombok.extern. slf4j.Slf4j;
import org. junit.Ignore;
import org .junit.Test;
import org. junit.runner.RunWith;
import org. springframework.beans.factory.annotation.Autowired;
import org. springframework.boot.test.context.SpringBootTest;
import org. springframework.test.context.junit4.SpringRunner;
import java. util.Date;
import java. util.List;
import static org.junit .Assert.*;
@RunWith (SpringRunner.class)
@SpringBootTest
@Slf4j
public class AreaDaoTest {
@Autowired
private AreaDao areaDao;
@Test
public void queryArea () throws Exception {
List <Area> areaList = areaDao.queryArea
();
// assertEquals (1, areaList.size());
log.info ("获取到的数据为:{}", areaList);
}
@Test
public void queryById () throws Exception {
Area area = areaDao.queryById(1);
log.info ("获取到的数据为:{}",area.toString());
}
@Test
// @Ignore
public void insertArea () throws Exception {
Area area = new Area ();
area.setAreaName ("南苑");
area.setPriority (1);
area.setCreateTime(new Date ());
area.setLastEditTime (new Date());
Integer result = areaDao.insertArea (area);
log.info ("插入完成的数据为:{}", result);
}
@Test
public void updateArea () throws Exception {
Area area = new Area ();
area.setAreaName ("东苑");
area.setLastEditTime (new Date());
area.setAreaId (1);
Integer result = areaDao.updateArea (area);
log.info ("更新完成后得到的结果为:{}", result);
}
@Test
public void deleteArea () throws Exception {
} |
|