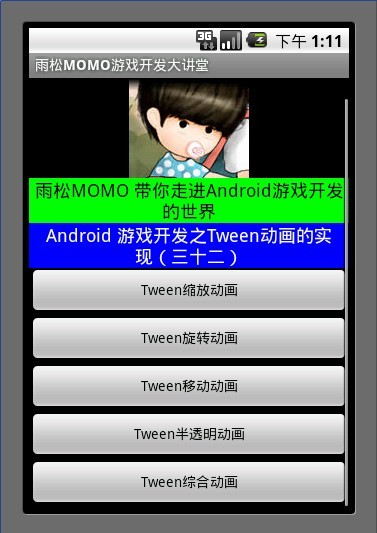
今天和大伙讨论一下Android开发中的Tween动画的实现。首先它和上一章我们讨论的Frame动画同属于系统提供的绘制动画的方法。Tween动画主要的功能是在绘制动画前设置动画绘制的轨迹,包括时间,
位置 ,等等。但是Tween动画的缺点是它只能设置起始点与结束点的两帧,中间过程全部由系统帮我们完成。所以在帧数比较多的游戏开发中是不太会用到它的。
Tween一共提供了4中动画的效果
- Scale:缩放动画
- Rotate:旋转动画
- Translate:移动动画
- Alpha::透明渐变动画
Tween与Frame动画类似都需要在res\anim路径下创建动画的
布局文件
补充:最近有盆友提问可不可以不用XML配置动画,希望可以在代码中配置。那MOMO当然要向大家补充了噢~~~
1.Scale缩放动画
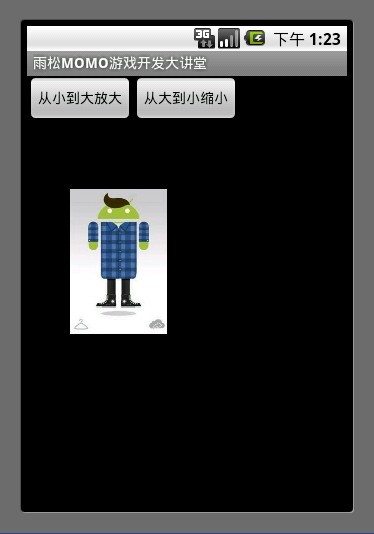
<scale>标签为缩放节点
android:fromXscale="1.0"
表示开始时X轴缩放比例为 1.0 (原图大小 * 1.0 为原图大小)
android:toXscale="0.0"表示结束时X轴缩放比例为0.0(原图大小
*0.0 为缩小到看不见)
android:fromYscale="1.0"
表示开始时Y轴缩放比例为 1.0 (原图大小 * 1.0 为原图大小)
android:toYscale="0.0"表示结束时Y轴缩放比例为0.0(原图大小
*0.0 为缩小的看不到了)
android:pivotX="50%" X轴缩放的位置为中心点
android:pivotY="50%" Y轴缩放的位置为中心点
android:duration="2000" 动画播放时间
这里是2000毫秒也就是2秒
这个动画布局设置动画从大到小进行缩小。
<?xml version="1.0" encoding="utf-8"?>
<scale xmlns:android="http://schemas.android.com/apk/res/android"
android:fromXScale="1.0"
android:toXScale="0.0"
android:fromYScale="1.0"
android:toYScale="0.0"
android:pivotX="50%"
android:pivotY="50%"
android:duration="2000">
</scale>
在代码中加载动画
mLitteAnimation = new ScaleAnimation(0.0f, 1.0f, 0.0f, 1.0f,
Animation.RELATIVE_TO_SELF, 0.5f,
Animation.RELATIVE_TO_SELF, 0.5f);
mLitteAnimation.setDuration(2000);
代码如下
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.ImageView;
public class ScaleActivity extends Activity {
/**缩小动画按钮**/
Button mButton0 = null;
/**放大动画按钮**/
Button mButton1 = null;
/**显示动画的ImageView**/
ImageView mImageView = null;
/**缩小动画**/
Animation mLitteAnimation = null;
/**放大动画**/
Animation mBigAnimation = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.scale);
/**拿到ImageView对象**/
mImageView = (ImageView)findViewById(R.id.imageView);
/**加载缩小与放大动画**/
mLitteAnimation = AnimationUtils.loadAnimation(this, R.anim.scalelitte);
mBigAnimation = AnimationUtils.loadAnimation(this, R.anim.scalebig);
mButton0 = (Button)findViewById(R.id.button0);
mButton0.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
/**播放缩小动画**/
mImageView.startAnimation(mLitteAnimation);
}
});
mButton1 = (Button)findViewById(R.id.button1);
mButton1.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
/**播放放大动画**/
mImageView.startAnimation(mBigAnimation);
}
});
}
}
2.Rotate旋转动画
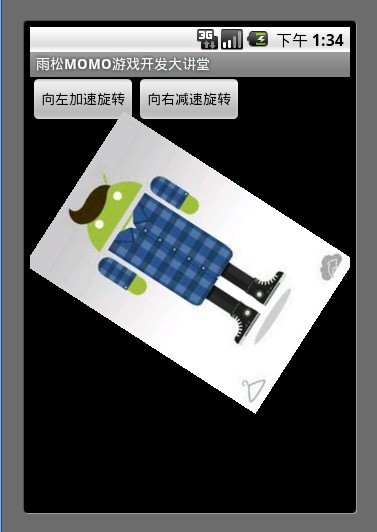
<rotate>标签为旋转节点
Tween一共为我们提供了3种动画渲染模式。
android:interpolator="@android:anim/accelerate_interpolator"
设置动画渲染器为加速动画(动画播放中越来越快)
android:interpolator="@android:anim/decelerate_interpolator"
设置动画渲染器为减速动画(动画播放中越来越慢)
android:interpolator="@android:anim/accelerate_decelerate_interpolator"
设置动画渲染器为先加速在减速(开始速度最快 逐渐减慢)
如果不写的话 默认为匀速运动
android:fromDegrees="+360"设置动画开始的角度
android:toDegrees="0"设置动画结束的角度
这个动画布局设置动画将向左做360度旋转加速运动。
<?xml version="1.0" encoding="utf-8"?>
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:interpolator="@android:anim/accelerate_interpolator"
android:fromDegrees="+360"
android:toDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:duration="2000"
/>
在代码中加载动画
mLeftAnimation = new RotateAnimation(360.0f, 0.0f,
Animation.RELATIVE_TO_SELF, 0.5f,
Animation.RELATIVE_TO_SELF, 0.5f);
mLeftAnimation.setDuration(2000);
代码实现
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.ImageView;
public class RotateActivity extends Activity {
/**向左旋转动画按钮**/
Button mButton0 = null;
/**向右旋转动画按钮**/
Button mButton1 = null;
/**显示动画的ImageView**/
ImageView mImageView = null;
/**向左旋转动画**/
Animation mLeftAnimation = null;
/**向右旋转动画**/
Animation mRightAnimation = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.retate);
/**拿到ImageView对象**/
mImageView = (ImageView)findViewById(R.id.imageView);
/**加载向左与向右旋转动画**/
mLeftAnimation = AnimationUtils.loadAnimation(this, R.anim.retateleft);
mRightAnimation = AnimationUtils.loadAnimation(this, R.anim.retateright);
mButton0 = (Button)findViewById(R.id.button0);
mButton0.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
/**播放向左旋转动画**/
mImageView.startAnimation(mLeftAnimation);
}
});
mButton1 = (Button)findViewById(R.id.button1);
mButton1.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
/**播放向右旋转动画**/
mImageView.startAnimation(mRightAnimation);
}
});
}
}
3.Translate移动动画
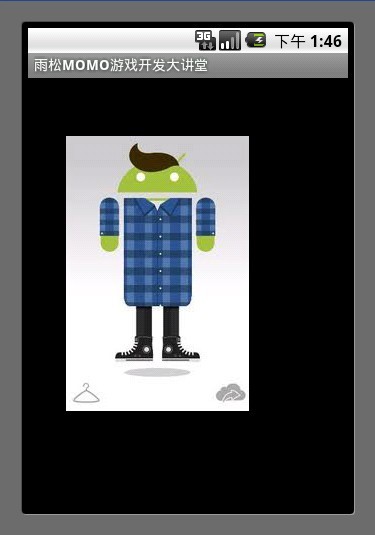
<translate>标签为移动节点
android:repeatCount="infinite"
设置动画为循环播放,这里可以写具体的int数值,设置动画播放几次,但是它记录次数是从0开始数的,比如这里设置为2
那么动画从0开始数数0 、1、 2 、实际上是播放了3次。
剩下的几个标签上面已经介绍过了。
这个动画布局设置动画从左到右(0.0),从上到下(320,480)做匀速移动。
<?xml version="1.0" encoding="utf-8"?>
<translate xmlns:android="http://schemas.android.com/apk/res/android"
android:fromXDelta="0"
android:toXDelta="320"
android:fromYDelta="0"
android:toYDelta="480"
android:duration="2000"
android:repeatCount="infinite"
/>
在代码中加载动画
mAnimation = new TranslateAnimation(0, 320, 0, 480);
mAnimation.setDuration(2000);
代码实现
import android.app.Activity;
import android.os.Bundle;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.ImageView;
public class TranslateActivity extends Activity {
/**显示动画的ImageView**/
ImageView mImageView = null;
/**移动动画**/
Animation mAnimation = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.translate);
/**拿到ImageView对象**/
mImageView = (ImageView)findViewById(R.id.imageView);
/**加载移动动画**/
mAnimation = AnimationUtils.loadAnimation(this, R.anim.translate);
/**播放移动动画**/
mImageView.startAnimation(mAnimation);
}
}
4 .Alpha:透明渐变动画
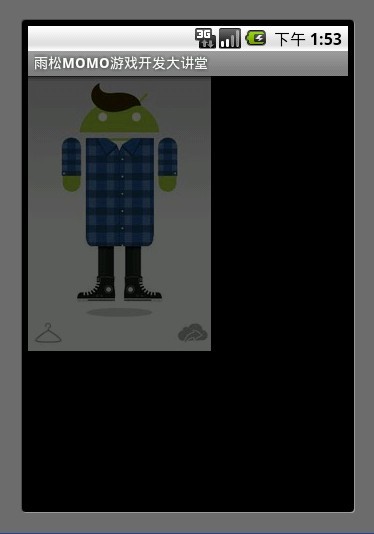
<alpha>标签为alpha透明度节点
android:fromAlpha="1.0" 设置动画起始透明度为1.0
表示完全不透明
android:toAlpha="0.0"设置动画结束透明度为0.0
表示完全透明
也就是说alpha的取值范围为0.0 - 1.0 之间
这个动画布局设置动画从完全不透明渐变到完全透明。
<?xml version="1.0" encoding="utf-8"?>
<alpha xmlns:android="http://schemas.android.com/apk/res/android"
android:fromAlpha="1.0"
android:toAlpha="0.0"
android:repeatCount="infinite"
android:duration="2000">
</alpha>
在代码中加载动画
mAnimation = new AlphaAnimation(1.0f, 0.0f);
mAnimation.setDuration(2000);
代码实现
import android.app.Activity;
import android.os.Bundle;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.ImageView;
public class AlphaActivity extends Activity {
/**显示动画的ImageView**/
ImageView mImageView = null;
/**透明动画**/
Animation mAnimation = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.translate);
/**拿到ImageView对象**/
mImageView = (ImageView)findViewById(R.id.imageView);
/**加载透明动画**/
mAnimation = AnimationUtils.loadAnimation(this, R.anim.alpha);
/**播放透明动画**/
mImageView.startAnimation(mAnimation);
}
}
5.综合动画
可以将上面介绍的4种动画设置在一起同时进行播放,那么就须要使用<set>标签将所有须要播放的动画放在一起。
这个动画布局设置动画同时播放移动、渐变、旋转。
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<rotate
android:interpolator="@android:anim/accelerate_interpolator"
android:fromDegrees="+360"
android:toDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:duration="2000"
android:repeatCount="infinite"
/>
<alpha android:fromAlpha="1.0"
android:toAlpha="0.0"
android:repeatCount="infinite"
android:duration="2000">
</alpha>
<translate
android:fromXDelta="0"
android:toXDelta="320"
android:fromYDelta="0"
android:toYDelta="480"
android:duration="2000"
android:repeatCount="infinite"
/>
</set>
代码实现
import android.app.Activity;
import android.os.Bundle;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.ImageView;
public class AllActivity extends Activity {
/**显示动画的ImageView**/
ImageView mImageView = null;
/**综合动画**/
Animation mAnimation = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.translate);
/**拿到ImageView对象**/
mImageView = (ImageView)findViewById(R.id.imageView);
/**加载综合动画**/
mAnimation = AnimationUtils.loadAnimation(this, R.anim.all);
/**播放综合动画**/
mImageView.startAnimation(mAnimation);
}
} |